使用MVVM
架构来开发APP的好处之一就是便于测试业务逻辑,由于ViewModel
不涉及界面,就不用考虑Mock对象了,测试起来也方便的多。
对ViewModel进行单元测试的一般流程
创建ViewModel
-> 把输入数据交给ViewModel
-> ViewModel
把输入数据进行业务逻辑处理,加工成输出数据 -> 测试ViewModel
的输出数据是否正确
进行单元测试前需做一些配置,可参考:
http://liuduoios.github.io/2015/10/24/Swift-2-%E4%B8%AD%E7%9A%84%E5%8D%95%E5%85%83%E6%B5%8B%E8%AF%95%E5%85%B3%E9%94%AE%E5%AD%97-testable/
下面拿一个简单的例子来说明
APP启动后界面如下:
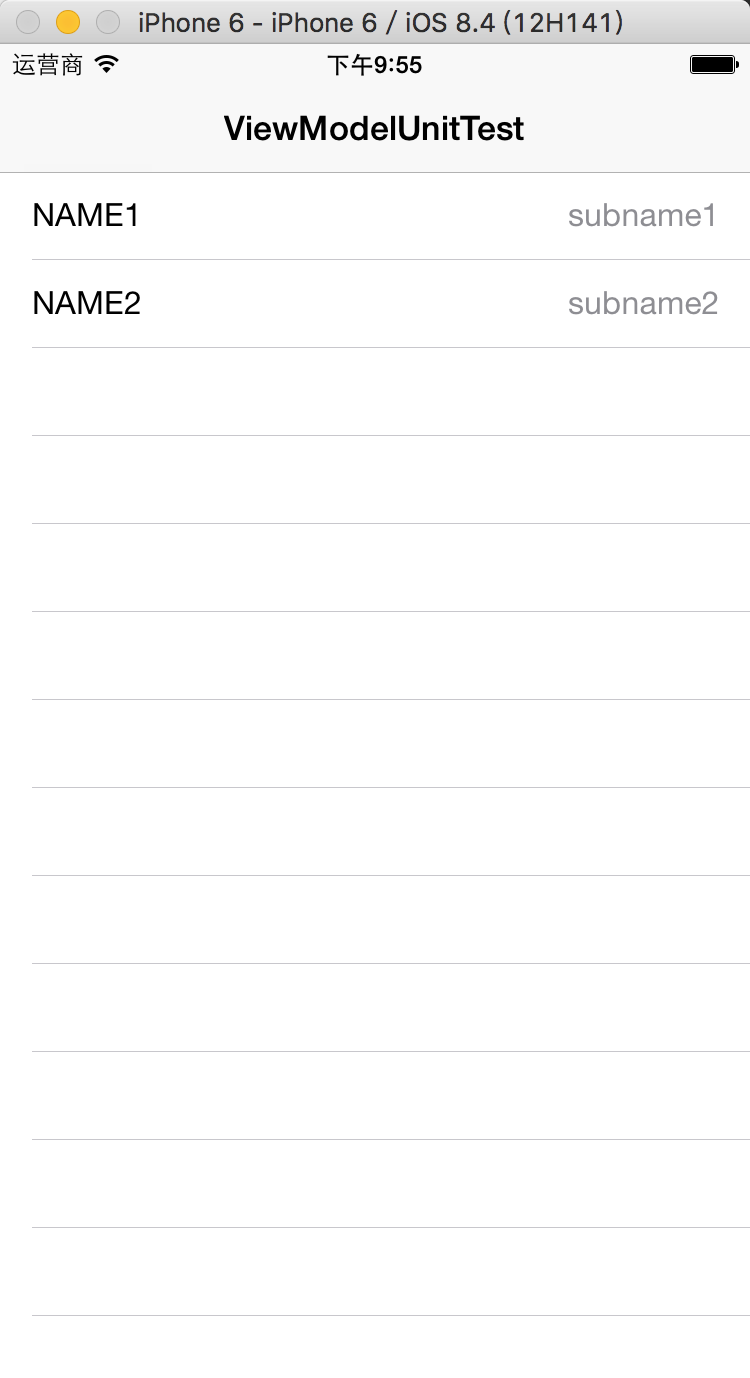
这个例子中含有两个ViewModel
类,一个是列表的ListViewModel
,其中含有根据接口返回数据来计算Cell行数的业务逻辑,内容如下:
这里数据绑定和变化监听用了开源库SwiftBond
https://github.com/SwiftBond/Bond
1 | import UIKit |
另一个是Cell的CellViewModel
,其中含有把title变为大写的业务逻辑,内容如下:
1 | import UIKit |
测试ListViewModel
这里设计到网络请求数据,因此要用到一个stub开源库,这里使用了Mockingjay
。
对于stub开源库的选择:
如果你的项目是纯Swift的,推荐使用Mockingjay
https://github.com/kylef/Mockingjay
如果你的项目是Objective-C或者OC和Swift混编的,推荐使用OHHTTPStubs
https://github.com/AliSoftware/OHHTTPStubs
1 | import XCTest |
测试CellViewModel
不涉及网络请求,直接对业务逻辑进行测试就好了。
1 | import XCTest |